Java Annotation Essentials, from Binary code point of view, with example of java.lang.Deprecated
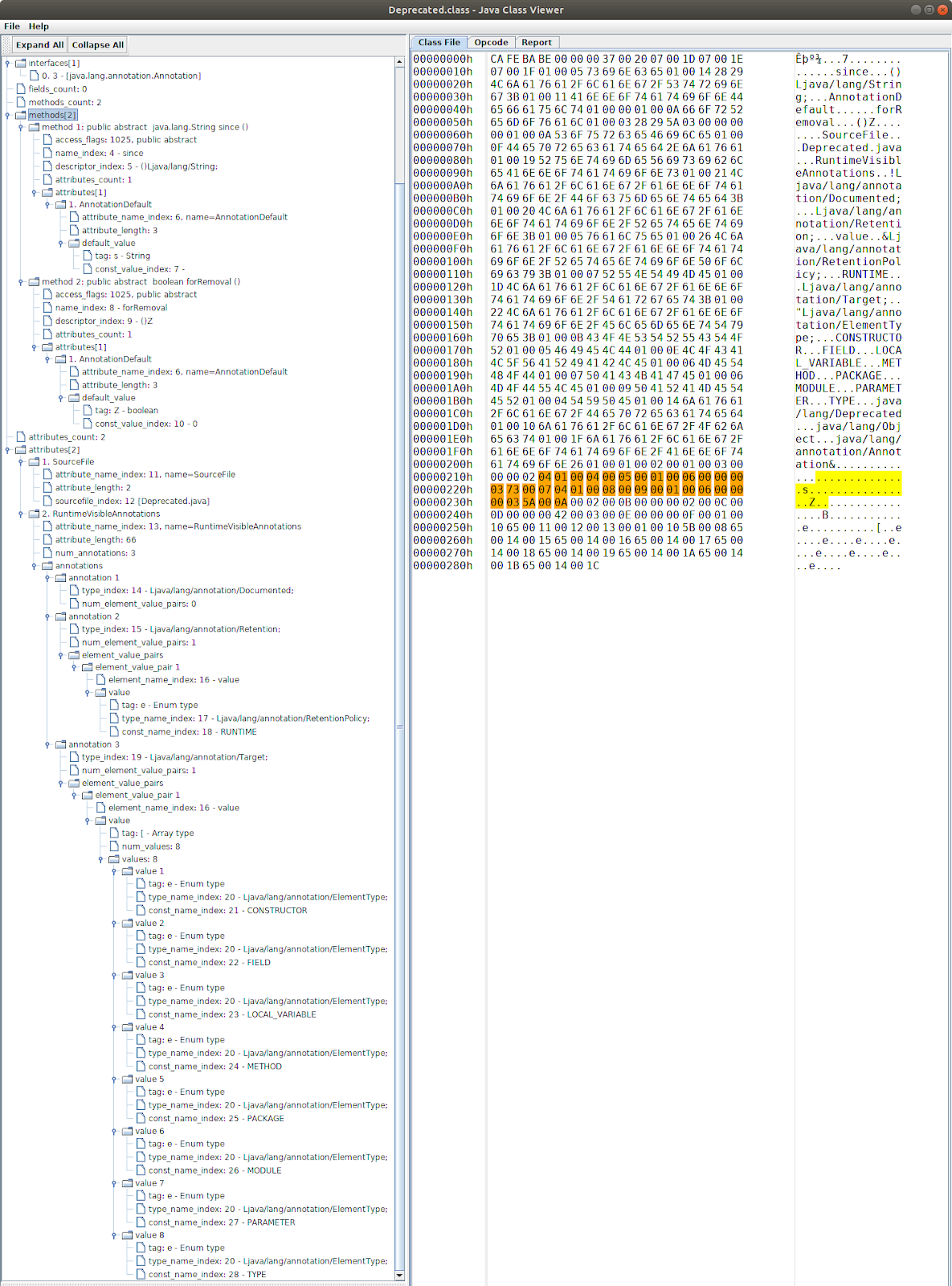
Java Annotations provides a mechanism to document and extend the code in Java programming language. Like we can annotation an API as deprecated , mapping the Database table and field to Java class and fields , define the REST API access points, asking JVM to do injections , etc. Binary Code is always the best document. Let's take an example from OpenJDK of the very popular annotation java.lang.Deprecated over the Java method java.lang . Class . newInstance() method. The Deprecated annotation is a widely used in Java source code, to a Java Object has been deprecated, and it could be removed in later release. Here is the source code of the Deprecated annotation: master/src/java.base/share/classes/java/lang/Deprecated.java 80 @Documented 81 @Retention(RetentionPolicy.RUNTIME) 82 @Target(value={CONSTRUCTOR, FIELD, LOCAL_VARIABLE, METHOD, PACKAGE, MODULE, PARAMETER, TYPE}) 83 public @interface Deprecated { 93 String since() default ""; 102 bool...